Learn about Natural Language Processing programming languages in this primer.
Table of Contents
ToggleNatural Language Processing or NLP is the branch of artificial intelligence that aims to facilitate communication between humans and machines by using natural human language as an interactive medium. It combines elements of data science, computing, and linguistics to develop systems and applications capable of interpreting, understanding, and acting upon natural language input in the form of spoken or written text.
Much of this activity requires coding and the construction of dedicated software architecture, so natural language processing programming exists as a specific field in the development arena.
Some Natural Language Processing Programming Languages
Semantic and syntax analysis forms a significant part of natural language processing, as does the development of NLP algorithms based on machine learning principles. Some of the core computing languages used in natural language processing have a data science and statistical analysis focus.
MATLAB, a fourth-generation programming language, and platform often used in representing and working with matrices. A high-performance technical computing language, MATLAB typically performs the mathematical computation and algorithm development underlying natural language processing operations.
The programming language R uses statistical methods and graphs to play a role in investigating big data, supporting NLP research and performing computationally intense learning analytics. A considerable number of natural language processing algorithms have been developed in R, making the language an ideal tool for NLP modeling and prototypes.
NLP Programming with Python
A lot of the coding activity in the natural language processing realm takes place in Python, an interpreted programming language with a syntax that often reads like standard English. With an ecosystem that actively supports the implementation of Artificial Intelligence (AI) and Machine Learning (ML) systems, Python also offers various libraries and other resources that facilitate NLP programming.
Chief among these is the Natural Language ToolKit (NLTK), the most popular library for natural language processing. It includes functions and data sets to support the most common techniques used in NLP, such as calculating how many times a particular word or token appears in a given piece of text (the FreqDist word frequency distribution class), extracting and tokenizing text data from HTML or XML files (the Beautiful Soup library), and performing sentiment analysis on blocks of text to determine whether the opinions expressed there are positive, negative, or neutral (VADER, or the Valence Aware Dictionary and sEntiment Reasoner).
NLP Programming with Java
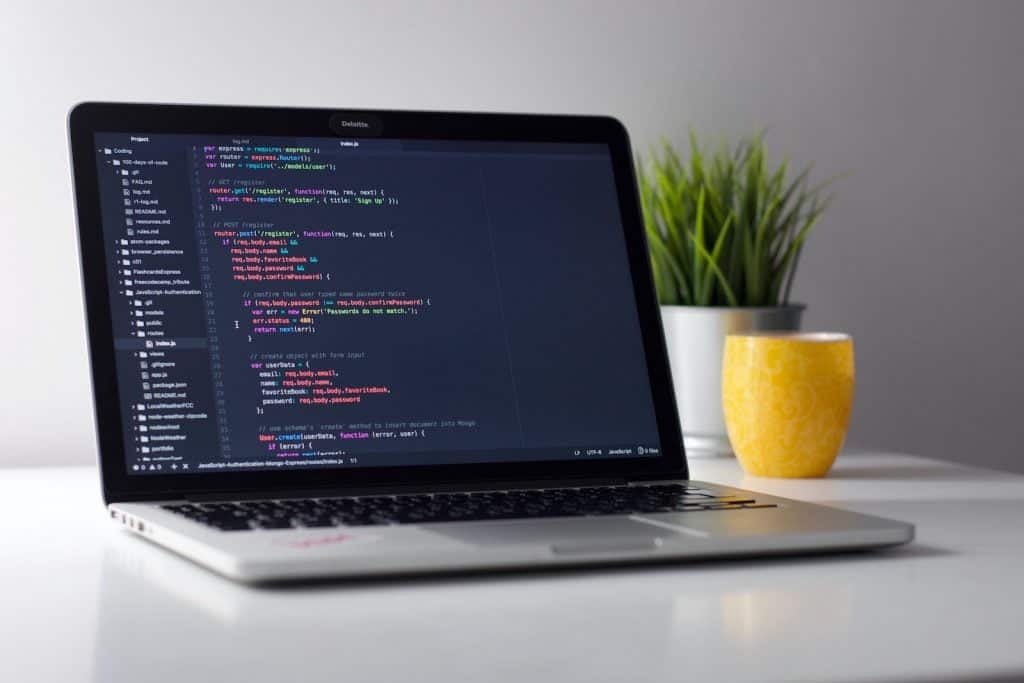
The most popular programming language for Android smartphones, Java is platform-independent and has an established history of facilitating conversational interfaces. Though natural language processing with Java can be a complex and challenging affair, successful NLP implementations in Java enable users to explore how to automatically organize text data using full-text search, clustering, tagging, and information extraction.
It’s possible to implement Natural Language Processing in a JavaScript project without struggling with integrating an external API (Application Programming Interface). You can do this with Cerebrum.js, an open-source package designed to perform advanced Machine Learning operations such as Natural Language Processing. Cerebrum.js enables programmers to process data within a JavaScript project, easing NLP implementation and reducing the cost and privacy risk of paying for an external API or transferring data to external servers.
Implementation of Cerebrum.js is typically a five-step process.
First, you have to install the Cerebrum.js package into your project, using the command:
npm i cerebrum.js
Next, you’ll need to import a selection of the pre-built Cerebrum.js functions that are relevant to your project. The syntax looks like this:
const Cerebrum = require(“cerebrum.js”);
const newCerebrum = new Cerebrum();
The third step is to create a data set for your natural language processing operation. Cerebrum.js takes an array of objects as its data set for NLP training and makes a learning model from this array. The array must contain at least three objects. Each object in the array has three characteristic properties: intent, utterances, and answers.
Intent is a parameter that’s used in grouping similar kinds of questions. Utterances consist of an array of strings that include all the questions in a particular intent category. And answers comprise the array of strings that include all the responses relevant to a specific intent.
The syntax for creating a data set in Cerebrum.js looks like this:
const dataset = [
{
intent: “agent.creator”,
utterances: [“who build me”, “who create me”],
answers: [
“You build me”,
“Its You”,
“You created me”
]
The fourth step in using Cerebrum.js is to train the data set using a built-in function called trainCerebrum(). To do this, you have to pass the data set as an argument to the trainCerebrum(dataSet) function. This function will return the string “Success” if training is successfully completed. Here’s some example syntax:
const train = async () => {
const response = await newCerebrum.trainCerebrum(dataset);
return response;
};
The fifth and final step is to call for a response from your model. The function cerebrumReplay() takes a string as an argument to pass the question that you wish to ask into the function, and the function then returns an appropriate answer for that question from the trained model.
The natural language processing java source code for eliciting this response looks like this:
const response = async (question) => {
const answer = await newCerebrum.cerebrumReplay(question);
return answer;
};
NLP with Node.js
Together with JavaScript, Node.js is gaining traction in the field of chatbots, where natural language processing enables conversational systems to better interpret user input, and natural language generation (NLG) can be employed to make the chatbot’s response seem more spontaneous and human.
In cases where the project is built with Node.js and the NLP that you’re using was written in another language, this introduces a new level of complexity. Fortunately, some resources can overcome this, such as an extensible dedicated natural language processing library and detection frameworks for node.js.
Several core techniques facilitate the implementation of node js natural language processing projects.
Rather than analyzing an entire text string, it’s easier and better to perform NLP methods on individual words in order to extract more data from them. The natural language processing technique of tokenization can accomplish this, and the Natural library for Node.js comes with a number of different tokenizers. For example, the syntax for the WordTokenizer looks like this:
var nlp = require(‘natural’);
var tokenizer = new nlp.WordTokenizer();
console.log(tokenizer.tokenize(“The Secret Designer: First Job Horror”));
In order to determine if two text strings match, Natural uses the Levenshtein distances algorithm, which checks for matches within the given context of the algorithm. It can be used for simple operations such as providing suggestions based on bad spelling:
var nlp = require(‘natural’);
console.log(natural.LevenshteinDistance(“Daine”,”Dane”));
The “String Distance” application of the Levenshtein distances algorithm is just one use case. Approximate String Matching is another implementation that you can apply to text strings with more context or with some text entity (a person’s name, city, country, etc.) that might be misspelled within a text string. And with suitable parameters, the algorithm may be applied for much more complex procedures.
For phonetics analysis, the metaphone.compare() method is highly efficient in identifying words that sound the same, but have a different meaning:
var nlp = require(‘natural’);
var metaphone = nlp.Metaphone;
if(metaphone.compare(‘see’, ‘sea’)) {
console.log(‘Phonetically they match!’);
}
One of the latest integrations in the Natural library is Wordnet, a dictionary database developed by Princeton University. The database enables Node.js programmers to look up words instantaneously, including all metadata associated with a word, such as synonyms, adjectives, and verbs.
In order to use Wordnet, you’ll have to install the wordnet-db NPM package by typing the following:
npm install wordnet-db
In conjunction with libraries like React, the Wordnet functionality could potentially enable natural language processing programmers to standardize the native dictionary facilities that most operating systems incorporate in their software and web browsers.